Python
Configure the Environment
-
Windows
- Enter the TA_CAN\python path in the terminal and execute
python install.py
- After successfully downloading, the following
- Enter the TA_CAN\python path in the terminal and execute
-
Linux
- Enter the TA_CAN\python path in the terminal and execute
python install.py
- After successfully downloading, the following
- Enter the TA_CAN\python path in the terminal and execute
Creating Class Objects
demo:
from motor_control import *
id = [0x01, 0x17]
port_name= "COM27" # Windows: "COM*" Linux: "/dev/ttyUSB*"
motor = MotorControl(port_name, id, 921600) # Params: serial port (str), list of actuator ID (0x05060100, 0x05061700)
Reset
reset(MotorControl object, flag) # The default value of flag is 0. When it is 1, more messages will be read
Phenomenon: The actuator LED turns red first and then blue
(Return actuator ID, serial number, firmware version)
from motor_control import *
id = [0x01, 0x17]
port_name= "COM27" # Windows: "COM*" Linux: "/dev/ttyUSB*"
motor = MotorControl(port_name, id, 921600) # Params: serial port (str), list of actuator ID (0x05060100, 0x05061700)
reset(motor)
Mode Selection
mode_selection(MotorControl object, <b>Control mode</b>,Feedback cycle 1, Feedback cycle 2, Feedback cycle 3)
(Print current operation mode)
Please refer to the figure below for Control mode
Control mode
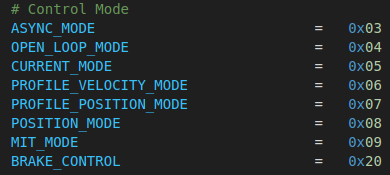
demo Enter Profile Velocity Mode
from motor_control import *
id = [0x01, 0x17] # list of int
duty_values = [500, 500] # list of int
port_name= "COM27" # Windows: "COM*" Linux: "/dev/ttyUSB*"
motor = MotorControl(port_name, id, 921600) # Params: serial port (str), list of actuator ID (0x05060100, 0x05061700)
mode_selection(motor, PROFILE_VELOCITY_MODE, 10, 10, 10) # Params: MotorControl object, operation mode, feedback cycle 1, 2, 3
ASYNC Mode
mode_selection(MotorControl object, ASYNC_MODE, xx, xx, xx)
write_async(MotorControl object, [Duty values])
(Try not to use it if there is no abnormality in the actuator)
demo: Set Duty Value to 500
from motor_control import *
id = [0x01, 0x17] # list of int
duty_values = [500, 500] # list of int
port_name= "COM27" # Windows: "COM*" Linux: "/dev/ttyUSB*"
motor = MotorControl(port_name, id, 921600) # Params: serial port (str), list of actuator ID (0x05060100, 0x05061700)
mode_selection(motor, ASYNC_MODE, 10, 10, 10) # Params: MotorControl object, operation mode, feedback cycle 1, 2, 3
write_async(motor, duty_values) # Params: MotorControl object, list of duty values
Open Loop Mode
mode_selection(MotorControl object, OPEN_LOOP_MODE, xx, xx, xx)
write_open_loop(MotorControl object, [Duty values])
(Try not to use it if there is no abnormality in the actuator)
demo: Set Duty Value to 500
from motor_control import *
id = [0x01, 0x17] # list of int
duty_values = [500, 500] # list of int
port_name = "COM27" # Windows: "COM*" Linux: "/dev/ttyUSB*"
motor = MotorControl(port_name, id, 921600) # Params: serial port (str), list of actuator ID (0x05060100, 0x05061700)
mode_selection(motor, OPEN_LOOP_MODE, 10, 10, 10) # Params: MotorControl object, operation mode, feedback cycle 1, 2, 3
write_open_loop(motor, duty_values) # Params: MotorControl object, list of duty values
Current Mode
mode_selection(MotorControl object, CURRENT_MODE, xx, xx, xx)
write_current(MotorControl object, [Target current])
demo: Set Target Current to 500mA
from motor_control import *
id = [0x01, 0x17] # list of int
cur = [500, 500] # list of int
port_name = "COM27" # Windows: "COM*" Linux: "/dev/ttyUSB*"
motor = MotorControl(port_name, id, 921600) # Params: serial port (str), list of actuator ID (0x05060100, 0x05061700)
mode_selection(motor, CURRENT_MODE, 10, 10, 10) # Params: MotorControl object, operation mode, feedback cycle 1, 2, 3
write_current(motor, cur) # Params: MotorControl object, list of target current
Profile Velocity Mode
mode_selection(MotorControl object, PROFILE_VELOCITY_MODE, xx, xx, xx)
write_profile_velocity(MotorControl object, [Current limit], [Target velocity])
demo: Set Target Velocity to 500rpm
from motor_control import *
id = [0x01, 0x17] # list of int
cur = [3000, 3000] # list of int
vel = [500, 500] # list of int
port_name = "COM27" # Windows: "COM*" Linux: "/dev/ttyUSB*"
motor = MotorControl(port_name, id, 921600) # Params: serial port (str), list of actuator ID (0x05060100, 0x05061700)
mode_selection(motor, PROFILE_VELOCITY_MODE, 10, 10, 10)# Params: MotorControl object, operation mode, feedback cycle 1, 2, 3
write_profile_velocity(motor, cur, vel) # Params: MotorControl object, list of current limit, list of target velocity
Profile Position Mode
mode_selection(MotorControl object, PROFILE_POSITION_MODE, xx, xx, xx)
write_profile_position(MotorControl object, [Current limit], [Velocity limit], [Target position])
demo: Set Target Position to 360°
from motor_control import *
id = [0x01, 0x17] # list of int
cur = [3000, 3000] # list of int
vel = [1000, 1000] # list of int
pos = [65535, 65535] # list of int
port_name = "COM27" # Windows: "COM*" Linux: "/dev/ttyUSB*"
motor = MotorControl(port_name, id, 921600) # Params: serial port (str), list of actuator ID (0x05060100, 0x05061700)
mode_selection(motor, PROFILE_POSITION_MODE, 10, 10, 10)# Params: MotorControl object, operation mode, feedback cycle 1, 2, 3
time.sleep(0.01)
write_profile_position(motor, cur, vel, pos) # Params: MotorControl object, list of current limit, list of velocity limit, list of target position
The value for one full rotation of the reducer end is 16 bits = 65536
Position Mode
mode_selection(MotorControl object, POSITION_MODE, xx, xx, xx)
write_position(MotorControl object, [Current limit], [Velocity limit], [Target position])
demo: Set Target Position to 360°
from motor_control import *
id = [0x01, 0x17] # list of int
cur = [3000, 3000] # list of int
vel = [1000, 1000] # list of int
pos = [65535, 65535] # list of int
port_name = "COM27" # Windows: "COM*" Linux: "/dev/ttyUSB*"
motor = MotorControl(port_name, id, 921600) # Params: serial port (str), list of actuator ID (0x05060100, 0x05061700)
mode_selection(motor, POSITION_MODE, 10, 10, 10)# Params: MotorControl object, operation mode, feedback cycle 1, 2, 3
time.sleep(0.01)
write_position(motor, cur, vel, pos) # Params: MotorControl object, list of current limit, list of velocity limit, list of target position
The value for one full rotation of the reducer end is 16 bits = 65536
MIT Mode
mode_selection(MotorControl object, MIT_MODE, xx, xx, xx)
write_mit(MotorControl object, [Target current], [Target velocity], [Target position], [KP], [KD])
demo: Point to point (The magnitude of the current should depend on the situation)
Target velocity is 0, others are not 0 (Current is feed-forward torque compensation)
from motor_control import *
id = [0x01, 0x17] # list of int
cur = [300, 300] # list of int
vel = [0, 0] # list of int
pos = [65535, 65535] # list of int
kp = [13.1, 13.1] # list of float
kd = [10.0, 10.0] # list of float
port_name = "COM27" # Windows: "COM*" Linux: "/dev/ttyUSB*"
motor = MotorControl(port_name, id, 921600) # Params: serial port (str), list of actuator ID (0x05060100, 0x05061700)
mode_selection(motor, MIT_MODE, 10, 10, 10) # Params: MotorControl object, operation mode, feedback cycle 1, 2, 3
time.sleep(0.01)
write_mit(motor, cur, vel, pos, kp, kd) # Params: MotorControl object, list of target current, list of target velocity, list of target position, list of KP, list of KD
Fixed velocity (The magnitude of the current should depend on the situation)
Target position and KP are 0, others are not 0 (Current is feed-forward torque compensation)
from motor_control import *
id = [0x01, 0x17] # list of int
cur = [300, 300] # list of int
vel = [500, 500] # list of int
pos = [0, 0] # list of int
kp = [0.0, 0.0] # list of float
kd = [10.0, 10.0] # list of float
port_name = "COM27" # Windows: "COM*" Linux: "/dev/ttyUSB*"
motor = MotorControl(port_name, id, 921600) # Params: serial port (str), list of actuator ID (0x05060100, 0x05061700)
mode_selection(motor, MIT_MODE, 10, 10, 10) # Params: MotorControl object, operation mode, feedback cycle 1, 2, 3
time.sleep(0.01)
write_mit(motor, cur, vel, pos, kp, kd) # Params: MotorControl object, list of target current, list of target velocity, list of target position, list of KP, list of KD
Fixed torque
Target current is not 0, others are 0
from motor_control import *
id = [0x01, 0x17] # list of int
cur = [500, 500] # list of int
vel = [0, 0] # list of int
pos = [0, 0] # list of int
kp = [0.0, 0.0] # list of float
kd = [0.0, 0.0] # list of float
port_name = "COM27" # Windows: "COM*" Linux: "/dev/ttyUSB*"
motor = MotorControl(port_name, id, 921600) # Params: serial port (str), list of actuator ID (0x05060100, 0x05061700)
mode_selection(motor, MIT_MODE, 10, 10, 10) # Params: MotorControl object, operation mode, feedback cycle 1, 2, 3
time.sleep(0.01)
write_mit(motor, cur, vel, pos, kp, kd) # Params: MotorControl object, list of target current, list of target velocity, list of target position, list of KP, list of KD
Read Parameters
results = get_v_p_c_v(MotorControl object)
(Return a list containing voltage, PWM, current, speed, and print "Parameter name: current value")
results = get_pos(MotorControl object)
(Return a list containing the motor position, actuator position, and prints "Parameter name: current value")
results = get_temp_error(MotorControl object)
(Return a list containing MOS tube temperature, motor temperature, G431 chip temperature, warning type and error type, and prints "Parameter name: current value")
Read voltage, PWM, current and velocity:
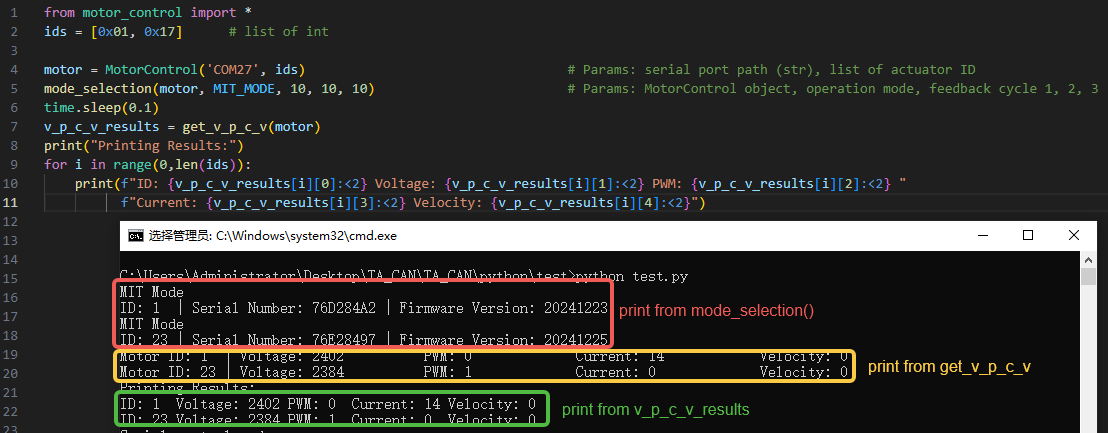
Read motor position and actuator position:
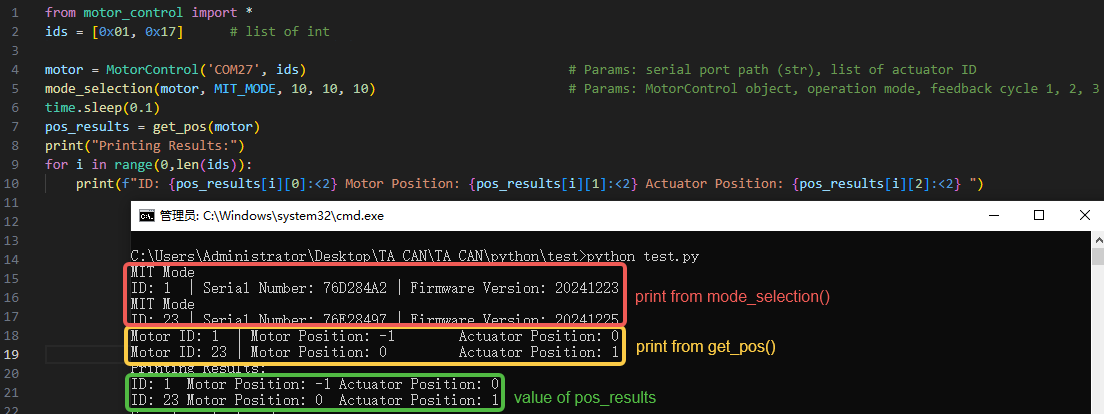
Read MOS tube temperature, motor temperature, G431 chip temperature, warning type and error type:
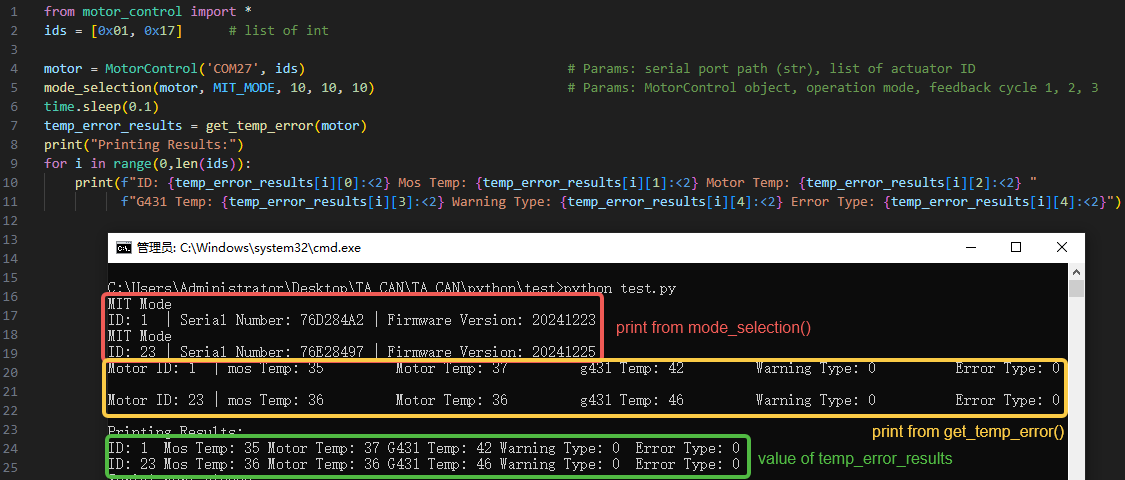
Other functions
brake, modify the acceleration and deceleration of the open-loop mode, modify the acceleration and deceleration of the profile speed, modify the current pid, modify the speed pid, modify the position pid:
from motor_control import *
id = [0x01, 0x17] # list of int
pwm = [-2000, -2000] # list of int
dur = [500, 500] # list of int
acc = [2000, 2000] # list of int
dec = [2000, 2000] # list of int
kp = [0.001, 0.001] # list of float
ki = [0.001, 0.001] # list of float
kd = [0.001, 0.001] # list of float
port_name = "COM27" # Windows: "COM*" Linux: "/dev/ttyUSB*"
motor = MotorControl(port_name, id, 921600) # Params: serial port (str), list of actuator ID (0x05060100, 0x05061700)
brake_control(motor, pwm, dur) # Params: MotorControl object, list of PWM, list of durations
openloop_acc_dec_set(motor, acc, dec) # Params: MotorControl object, list of acceleration, list of deceleration
velocity_acc_dec_set(motor, acc, dec) # Params: MotorControl object, list of acceleration, list of deceleration
current_pid_set(motor, kp, ki) # Params: MotorControl object, list of KP, list of KI
velocity_pid_set(motor, kp, ki) # Params: MotorControl object, list of KP, list of KI
position_pid_set(motor, kp, kd) # Params: MotorControl object, list of KP, list of KD
Modify the acceleration and deceleration of the contour speed in real time:
from motor_control import *
acc_dec = 0
id = [0x01, 0x17] # list of int
cur = [3000, 3000] # list of int
vel = [1000, 1000] # list of int
vel_0 = [0, 0] # list of int
port_name = "COM27" # Windows: "COM*" Linux: "/dev/ttyUSB*"
motor = MotorControl(port_name, id, 921600) # Params: serial port (str), list of actuator ID (0x05060100, 0x05061700)
mode_selection(motor, PROFILE_VELOCITY_MODE, 10, 10, 10) # Params: MotorControl object, operation mode, feedback cycle 1, 2, 3
time.sleep(0.01)
while 1:
acc_dec += 100
acc = [acc_dec, acc_dec]
dec = [acc_dec, acc_dec]
velocity_acc_dec_set(motor, acc, dec) # Params: MotorControl object, list of acceleration, list of deceleration
print(acc_dec)
write_profile_velocity(motor, cur, vel) # Params: MotorControl object, list of current limit, list of target velocity
time.sleep(3)
write_profile_velocity(motor, cur, vel_0) # Params: MotorControl object, list of current limit, list of target velocity
time.sleep(4)
if acc_dec >= 2000:
break