Python
您可以选择观看视频或者查看文档,这两种方式所讲的内容几乎是相同的:
配置环境
-
Windows
- 在终端进入TA_CAN\python路径,执行
python install.py
- 下载成功后如下
- 在终端进入TA_CAN\python路径,执行
-
Linux
- 在终端进入TA_CAN\python路径,执行
python install.py
- 下载成功后如下
- 在终端进入TA_CAN\python路径,执行
创建类对象
demo:
from motor_control import *
id = [0x01, 0x17]
port_name= "COM27" # Windows: "COM*" Linux: "/dev/ttyUSB*"
motor = MotorControl(port_name, id, 921600) # Params: serial port (str), list of actuator ID (0x05060100, 0x05061700)
复位
reset(MotorControl object,flag) # flag默认值为0,当为1时表示读取更多消息。
现象:执行器LED灯先变红后变蓝
(返回执行器ID,序列号,固件版本)
from motor_control import *
id = [0x01, 0x17]
port_name= "COM27" # Windows: "COM*" Linux: "/dev/ttyUSB*"
motor = MotorControl(port_name, id, 921600) # Params: serial port (str), list of actuator ID (0x05060100, 0x05061700)
reset(motor)
模式选择
mode_selection(MotorControl object, <b>Control mode</b>,Feedback cycle 1, Feedback cycle 2, Feedback cycle 3)
(打印当前操作模式)
❗
控制模式的类别请参考下图
控制模式
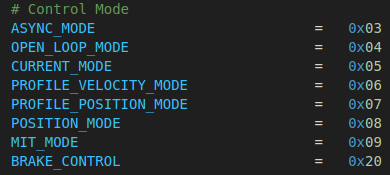
demo 进入轮廓速度模式
from motor_control import *
id = [0x01, 0x17] # list of int
duty_values = [500, 500] # list of int
port_name= "COM27" # Windows: "COM*" Linux: "/dev/ttyUSB*"
motor = MotorControl(port_name, id, 921600) # Params: serial port (str), list of actuator ID (0x05060100, 0x05061700)
mode_selection(motor, PROFILE_VELOCITY_MODE, 10, 10, 10) # Params: MotorControl object, operation mode, feedback cycle 1, 2, 3
异步模式
mode_selection(MotorControl object, ASYNC_MODE, xx, xx, xx)
write_async(MotorControl object, [Duty values])
(执行器无异常时尽量不使用)
demo: 设置占空值为500
from motor_control import *
id = [0x01, 0x17] # list of int
duty_values = [500, 500] # list of int
port_name= "COM27" # Windows: "COM*" Linux: "/dev/ttyUSB*"
motor = MotorControl(port_name, id, 921600) # Params: serial port (str), list of actuator ID (0x05060100, 0x05061700)
mode_selection(motor, ASYNC_MODE, 10, 10, 10) # Params: MotorControl object, operation mode, feedback cycle 1, 2, 3
write_async(motor, duty_values) # Params: MotorControl object, list of duty values
开环模式
mode_selection(MotorControl object, OPEN_LOOP_MODE, xx, xx, xx)
write_open_loop(MotorControl object, [Duty values])
(执行器无异常时尽量不使用)
demo: 设置占空值为500
from motor_control import *
id = [0x01, 0x17] # list of int
duty_values = [500, 500] # list of int
port_name = "COM27" # Windows: "COM*" Linux: "/dev/ttyUSB*"
motor = MotorControl(port_name, id, 921600) # Params: serial port (str), list of actuator ID (0x05060100, 0x05061700)
mode_selection(motor, OPEN_LOOP_MODE, 10, 10, 10) # Params: MotorControl object, operation mode, feedback cycle 1, 2, 3
write_open_loop(motor, duty_values) # Params: MotorControl object, list of duty values
电流模式
mode_selection(MotorControl object, CURRENT_MODE, xx, xx, xx)
write_current(MotorControl object, [Target current])
demo: 将目标电流设置成500mA
from motor_control import *
id = [0x01, 0x17] # list of int
cur = [500, 500] # list of int
port_name = "COM27" # Windows: "COM*" Linux: "/dev/ttyUSB*"
motor = MotorControl(port_name, id, 921600) # Params: serial port (str), list of actuator ID (0x05060100, 0x05061700)
mode_selection(motor, CURRENT_MODE, 10, 10, 10) # Params: MotorControl object, operation mode, feedback cycle 1, 2, 3
write_current(motor, cur) # Params: MotorControl object, list of target current
轮廓速度模式
mode_selection(MotorControl object, PROFILE_VELOCITY_MODE, xx, xx, xx)
write_profile_velocity(MotorControl object, [Current limit], [Target velocity])
demo: 将目标速度设置成500rpm
from motor_control import *
id = [0x01, 0x17] # list of int
cur = [3000, 3000] # list of int
vel = [500, 500] # list of int
port_name = "COM27" # Windows: "COM*" Linux: "/dev/ttyUSB*"
motor = MotorControl(port_name, id, 921600) # Params: serial port (str), list of actuator ID (0x05060100, 0x05061700)
mode_selection(motor, PROFILE_VELOCITY_MODE, 10, 10, 10)# Params: MotorControl object, operation mode, feedback cycle 1, 2, 3
write_profile_velocity(motor, cur, vel) # Params: MotorControl object, list of current limit, list of target velocity
轮廓位置模式
mode_selection(MotorControl object, PROFILE_POSITION_MODE, xx, xx, xx)
write_profile_position(MotorControl object, [Current limit], [Velocity limit], [Target position])
demo: 将目标位置设置成360°
from motor_control import *
id = [0x01, 0x17] # list of int
cur = [3000, 3000] # list of int
vel = [1000, 1000] # list of int
pos = [65535, 65535] # list of int
port_name = "COM27" # Windows: "COM*" Linux: "/dev/ttyUSB*"
motor = MotorControl(port_name, id, 921600) # Params: serial port (str), list of actuator ID (0x05060100, 0x05061700)
mode_selection(motor, PROFILE_POSITION_MODE, 10, 10, 10)# Params: MotorControl object, operation mode, feedback cycle 1, 2, 3
time.sleep(0.01)
write_profile_position(motor, cur, vel, pos) # Params: MotorControl object, list of current limit, list of velocity limit, list of target position
❗
减速机端旋转一圈的值为16bits=65535
位置模式
mode_selection(MotorControl object, POSITION_MODE, xx, xx, xx)
write_position(MotorControl object, [Current limit], [Velocity limit], [Target position])
demo: 将目标位置设置成360°
from motor_control import *
id = [0x01, 0x17] # list of int
cur = [3000, 3000] # list of int
vel = [1000, 1000] # list of int
pos = [65535, 65535] # list of int
port_name = "COM27" # Windows: "COM*" Linux: "/dev/ttyUSB*"
motor = MotorControl(port_name, id, 921600) # Params: serial port (str), list of actuator ID (0x05060100, 0x05061700)
mode_selection(motor, POSITION_MODE, 10, 10, 10)# Params: MotorControl object, operation mode, feedback cycle 1, 2, 3
time.sleep(0.01)
write_position(motor, cur, vel, pos) # Params: MotorControl object, list of current limit, list of velocity limit, list of target position
❗
减速机端旋转一圈的值为16bits=65536
MIT模式
mode_selection(MotorControl object, MIT_MODE, xx, xx, xx)
write_mit(MotorControl object, [Target current], [Target velocity], [Target position], [KP], [KD])
demo: 点到点(电流的大小应视情况而定)
❗
目标速度为0,其他不为0 (电流为前馈转矩补偿)
from motor_control import *
id = [0x01, 0x17] # list of int
cur = [300, 300] # list of int
vel = [0, 0] # list of int
pos = [65535, 65535] # list of int
kp = [13.1, 13.1] # list of float
kd = [10.0, 10.0] # list of float
port_name = "COM27" # Windows: "COM*" Linux: "/dev/ttyUSB*"
motor = MotorControl(port_name, id, 921600) # Params: serial port (str), list of actuator ID (0x05060100, 0x05061700)
mode_selection(motor, MIT_MODE, 10, 10, 10) # Params: MotorControl object, operation mode, feedback cycle 1, 2, 3
time.sleep(0.01)
write_mit(motor, cur, vel, pos, kp, kd) # Params: MotorControl object, list of target current, list of target velocity, list of target position, list of KP, list of KD
固定速度(电流的大小应视情况而定)
❗
目标位置和KP为0,其他不为0 (电流为前馈转矩补偿)
from motor_control import *
id = [0x01, 0x17] # list of int
cur = [300, 300] # list of int
vel = [500, 500] # list of int
pos = [0, 0] # list of int
kp = [0.0, 0.0] # list of float
kd = [10.0, 10.0] # list of float
port_name = "COM27" # Windows: "COM*" Linux: "/dev/ttyUSB*"
motor = MotorControl(port_name, id, 921600) # Params: serial port (str), list of actuator ID (0x05060100, 0x05061700)
mode_selection(motor, MIT_MODE, 10, 10, 10) # Params: MotorControl object, operation mode, feedback cycle 1, 2, 3
time.sleep(0.01)
write_mit(motor, cur, vel, pos, kp, kd) # Params: MotorControl object, list of target current, list of target velocity, list of target position, list of KP, list of KD
固定扭矩
❗
目标电流不为0,其他都为0
from motor_control import *
id = [0x01, 0x17] # list of int
cur = [500, 500] # list of int
vel = [0, 0] # list of int
pos = [0, 0] # list of int
kp = [0.0, 0.0] # list of float
kd = [0.0, 0.0] # list of float
port_name = "COM27" # Windows: "COM*" Linux: "/dev/ttyUSB*"
motor = MotorControl(port_name, id, 921600) # Params: serial port (str), list of actuator ID (0x05060100, 0x05061700)
mode_selection(motor, MIT_MODE, 10, 10, 10) # Params: MotorControl object, operation mode, feedback cycle 1, 2, 3
time.sleep(0.01)
write_mit(motor, cur, vel, pos, kp, kd) # Params: MotorControl object, list of target current, list of target velocity, list of target position, list of KP, list of KD
读取参数
results = get_v_p_c_v(MotorControl object)
(返回一个包含电压、PWM、电流、速度的列表,打印"参数名: 当前值")
results = get_pos(MotorControl object)
(返回一个包含电机位置、执行器位置的列表,打印"参数名: 当前值")
results = get_temp_error(MotorControl object)
(返回一个包含mos管温度、电机温度、G431芯片温度、警告类型和错误类型的列表,打印"参数名: 当前值")
读电压、PWM、电流和速度
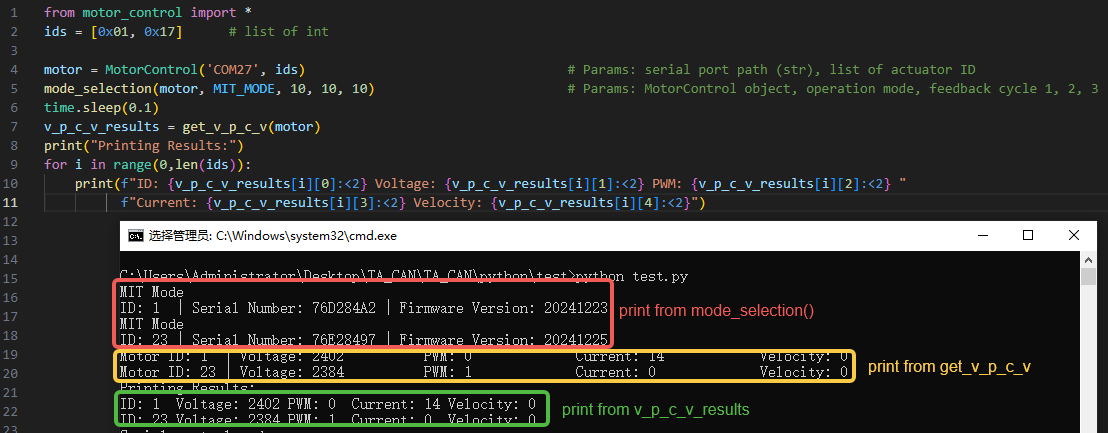
读电机位置和执行器位置
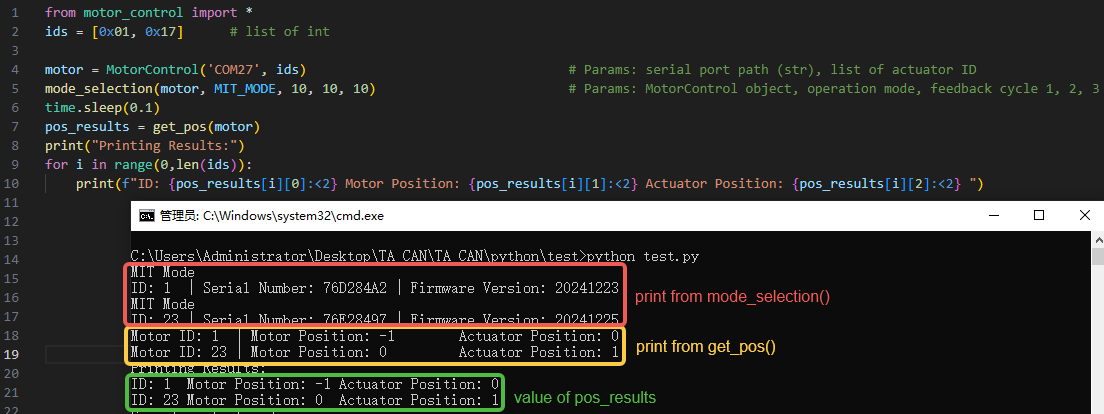
读mos管温度、电机温度、G431芯片温度、警告类型和错误类型
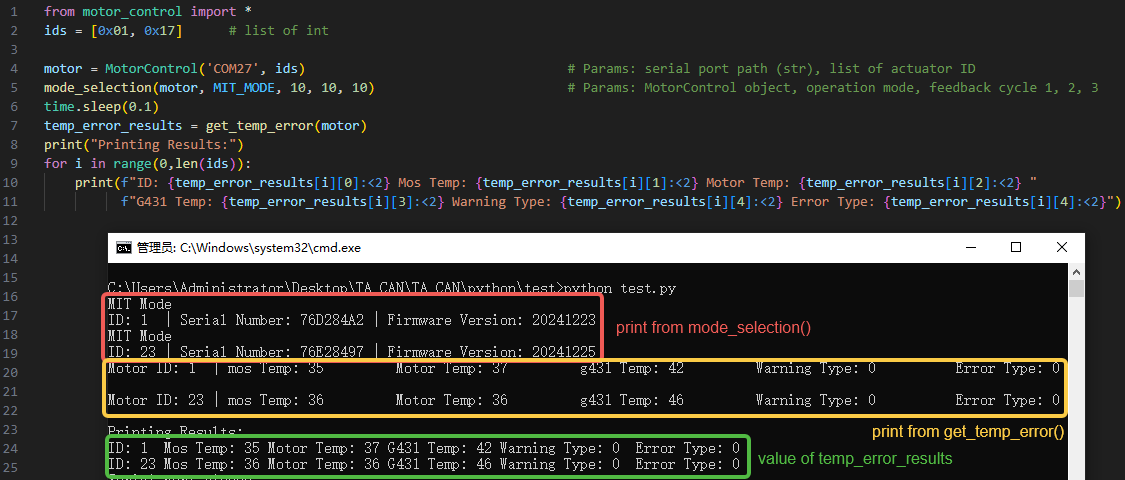
其他功能
刹车、修改开环模式加减速度、修改profile速度加减速度、修改电流pid、修改速度pid、修改位置pid
from motor_control import *
id = [0x01, 0x17] # list of int
pwm = [-2000, -2000] # list of int
dur = [500, 500] # list of int
acc = [2000, 2000] # list of int
dec = [2000, 2000] # list of int
kp = [0.001, 0.001] # list of float
ki = [0.001, 0.001] # list of float
kd = [0.001, 0.001] # list of float
port_name = "COM27" # Windows: "COM*" Linux: "/dev/ttyUSB*"
motor = MotorControl(port_name, id, 921600) # Params: serial port (str), list of actuator ID (0x05060100, 0x05061700)
brake_control(motor, pwm, dur) # Params: MotorControl object, list of PWM, list of durations
openloop_acc_dec_set(motor, acc, dec) # Params: MotorControl object, list of acceleration, list of deceleration
velocity_acc_dec_set(motor, acc, dec) # Params: MotorControl object, list of acceleration, list of deceleration
current_pid_set(motor, kp, ki) # Params: MotorControl object, list of KP, list of KI
velocity_pid_set(motor, kp, ki) # Params: MotorControl object, list of KP, list of KI
position_pid_set(motor, kp, kd) # Params: MotorControl object, list of KP, list of KD
实时修改轮廓速度的加速度和减速度
from motor_control import *
acc_dec = 0
id = [0x01, 0x17] # list of int
cur = [3000, 3000] # list of int
vel = [1000, 1000] # list of int
vel_0 = [0, 0] # list of int
port_name = "COM27" # Windows: "COM*" Linux: "/dev/ttyUSB*"
motor = MotorControl(port_name, id, 921600) # Params: serial port (str), list of actuator ID (0x05060100, 0x05061700)
mode_selection(motor, PROFILE_VELOCITY_MODE, 10, 10, 10) # Params: MotorControl object, operation mode, feedback cycle 1, 2, 3
time.sleep(0.01)
while 1:
acc_dec += 100
acc = [acc_dec, acc_dec]
dec = [acc_dec, acc_dec]
velocity_acc_dec_set(motor, acc, dec) # Params: MotorControl object, list of acceleration, list of deceleration
print(acc_dec)
write_profile_velocity(motor, cur, vel) # Params: MotorControl object, list of current limit, list of target velocity
time.sleep(3)
write_profile_velocity(motor, cur, vel_0) # Params: MotorControl object, list of current limit, list of target velocity
time.sleep(4)
if acc_dec >= 2000:
break