Using-MIT-mode
MIT Model Description:
The following figure is the control block diagram of the actuator MIT mode:
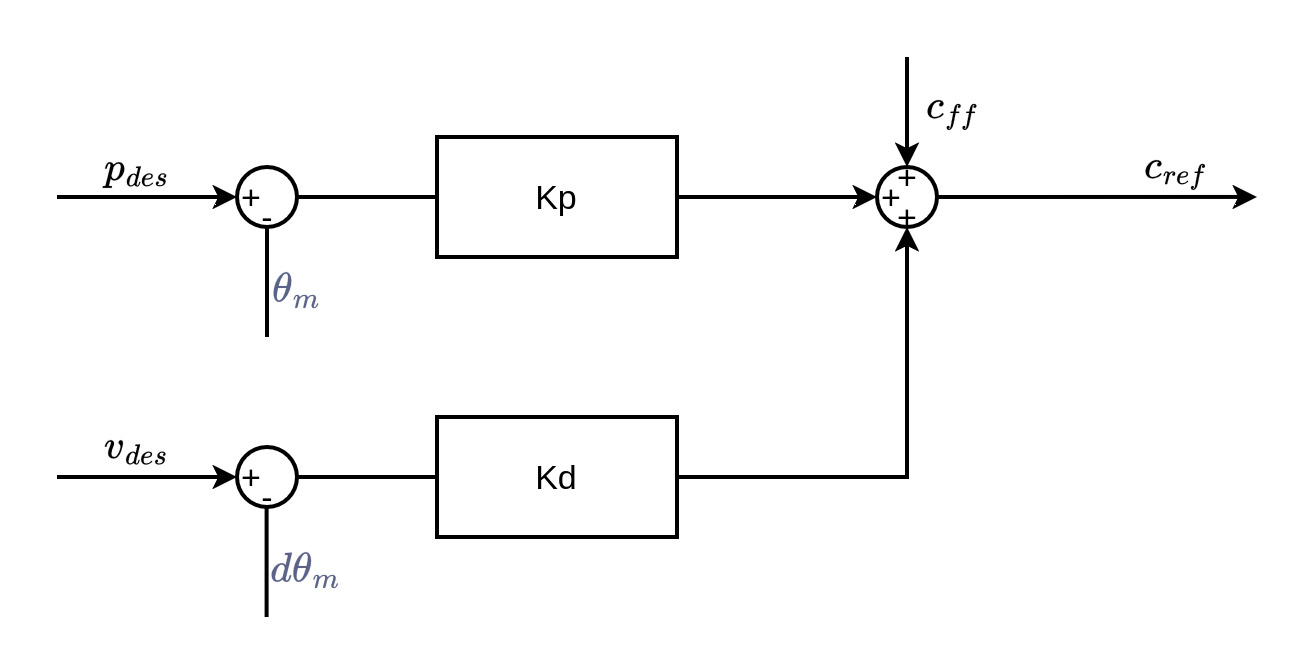
Figure 1 MIT mode control block diagram
MIT mode is a control method developed by MIT for precise torque control. It can achieve mixed control of current, speed and position. The calculation formula is as follows:
In the above formula, the output values of the speed loop and the position loop are added to the feedforward current to obtain the reference current, where:
is the expected position of the actuator output shaft, in counts;
is the current position of the actuator output shaft, in counts; is the expected speed of the reducer input, in rpm;
is the current speed of the reducer input, in rpm;
In this mode, there are 5 control parameters, which need to be sent in two CAN frames. One frame is the motor current, speed, and reducer position information (which can be modified to the position of the motor end in the motor parameter setting), and the other is Kp and Kd. For the message content, please refer to the communication protocol introduction. Reasonable settings of kp, kd and current values can effectively control the stiffness, damping and torque of the actuator rotation.
example:
- When the kp and kd values are both 0, the rotational stiffness and damping are both 0, which is equivalent to the current mode, and the phase current can be directly controlled:
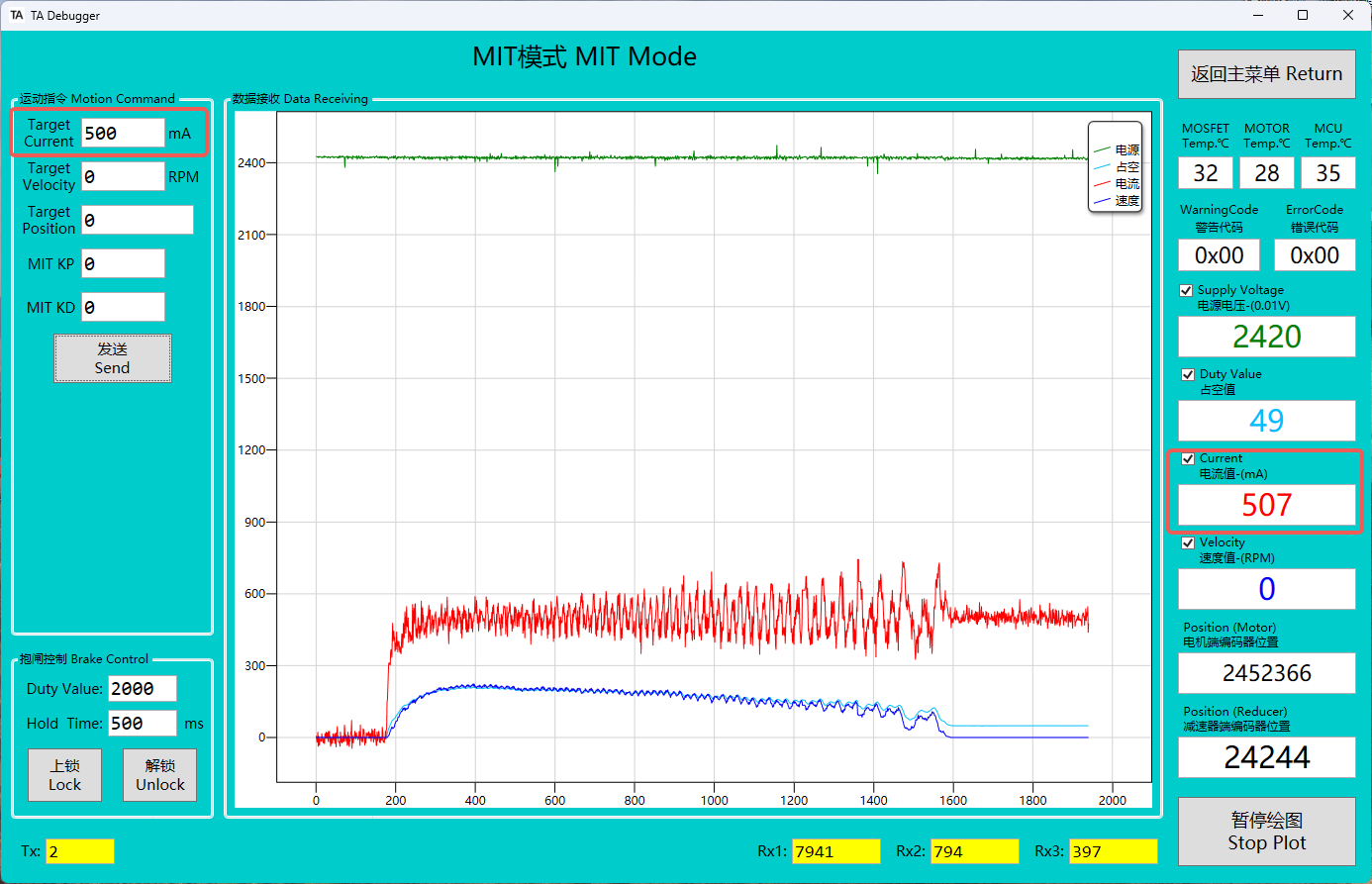
- When the kp value is 0 and the kd value is not 0, the stiffness is 0, the damping is not 0, and the input current (feedforward torque compensation) and speed can control the rotation speed of the actuator:
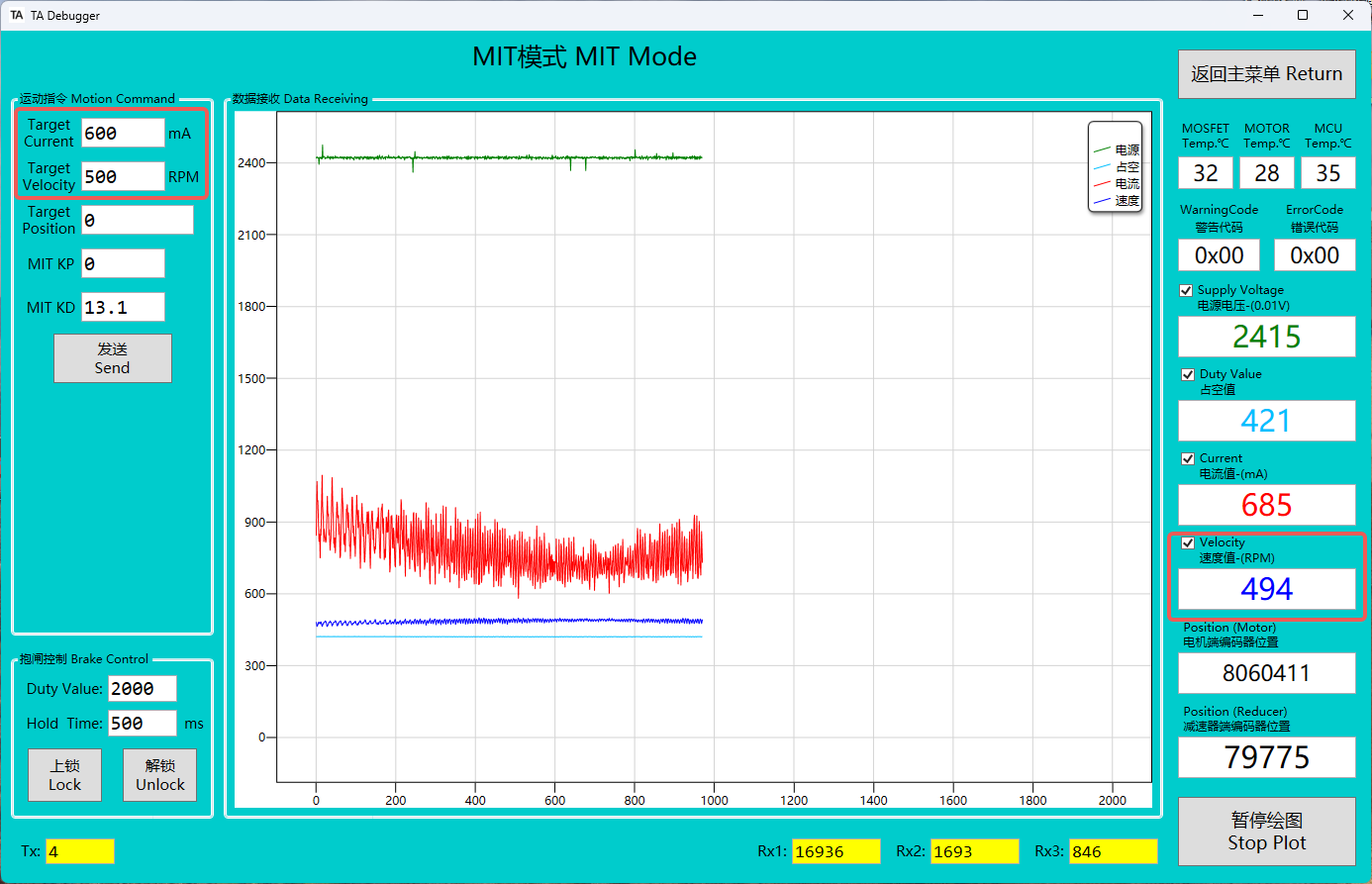
- When the values of kp and kd are not 0, there are many cases. Here are two of them:
(1) When the speed is 0, the input current (feedforward torque compensation) and position can achieve fixed-point control, as shown in the figure:
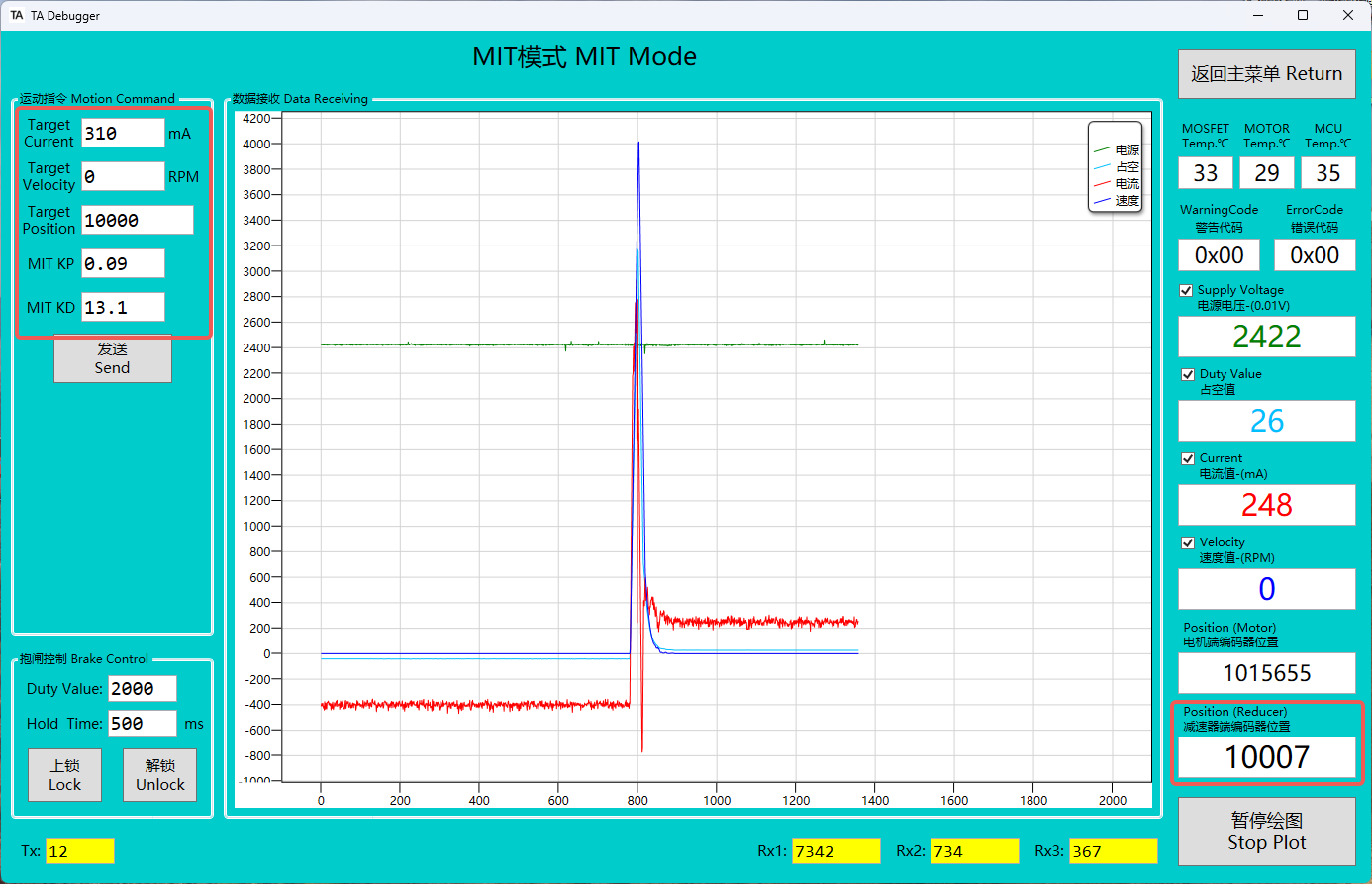
(2) When the desired position is a continuous differentiable function that changes with time, is the derivative of, which can realize position and speed tracking and realize the function of rotating the desired angle at the desired speed. The following figure is a test curve diagram of the target trajectory and the actual trajectory:
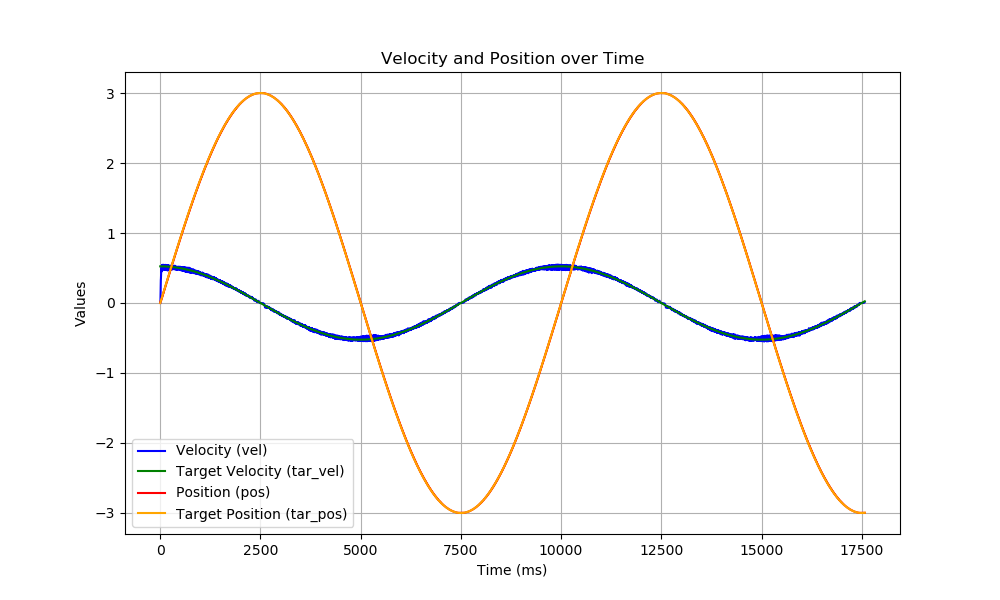
When kp is not 0 and kd is 0, the damping of the actuator is 0. At this time, the actuator will oscillate around the target position. This is not recommended.
SDK:
C++
- Single actuator
motor.mode_selection(Mode::MIT_MODE, xx, xx, xx)
motor.write_mit(Target current, Target velocity, Target position, KP, KD)
demo Fixed current
The target current is not 0, and the others are 0
#include <motor_control.h>
string port_name = "COM27" // Windows: "COM*" Linux: "/dev/ttyUSB*"
MotorControl motor(port_name, 921600, 0x01); // Params: serial port path (string), baud rate (uint32_t), actuator id (0x05060100)
int main()
{
motor.mode_selection(Mode::MIT_MODE, 10, 10, 10); // Params: Mode::operation mode, feedback cycle 1, 2, 3
Sleep(10); // Windows: Sleep(ms) Linux: sleep(s)
motor.write_mit(500, 0, 0, 0.0, 0.0); // Params: target current, target velocity, target position, kp, kd
}
Fixed speed (the current should be determined according to the situation)
The target position and KP are 0, and the others are not 0
#include <motor_control.h>
string port_name = "COM27"; // Windows: "COM*" Linux: "/dev/ttyUSB*"
MotorControl motor(port_name, 921600, 0x01); // Params: serial port path (string), baud rate (uint32_t), actuator id (0x05060100)
int main()
{
motor.mode_selection(Mode::MIT_MODE, 10, 10, 10); // Params: Mode::operation mode, feedback cycle 1, 2, 3
Sleep(10); // Windows: Sleep(ms) Linux: sleep(s)
motor.write_mit(300, 500, 0, 0.0, 10.0); // Params: target current, target velocity, target position, kp, kd
}
Point to point (the current should be determined according to the situation)
The target speed is 0, and the others are not 0
#include <motor_control.h>
string port_name = "COM27"; // Windows: "COM*" Linux: "/dev/ttyUSB*"
MotorControl motor(port_name, 921600, 0x01); // Params: serial port path (string), baud rate (uint32_t), actuator id (0x05060100)
int main()
{
motor.mode_selection(Mode::MIT_MODE, 10, 10, 10); // Params: Mode::operation mode, feedback cycle 1, 2, 3
Sleep(10); // Windows: Sleep(ms) Linux: sleep(s)
motor.write_mit(300, 0, 65535, 13.1, 10.0); // Params: target current, target velocity, target position, kp, kd
}
- Multiple actuators
motors.mode_selection(Mode::MIT_MODE, xx, xx, xx)
motors.write_mit(vector<int16_t>target current, vector<int16_t>target velocity, vector<int16_t>target position, vector<float>KP, vector<float>KD)
demo: 固定电流
目标电流不为0,其他都为0
#include <multi_motor_control.h>
vector<int16_t>cur = { 500, 500 };
vector<int16_t>vel = { 0, 0 };
vector<int32_t>pos = { 0, 0 };
vector<float>kp = { 0.0, 0.0 };
//vector<float>kd = { 0.0, 0.0 };
string port_name = "COM27"; // Windows: "COM*" Linux: "/dev/ttyUSB*"
MultiMotorControl motors(port_name, 921600, ids); // Params: serial port path (string), baud rate (uint32_t), actuator ids (0x05060100, 0x05061700)
int main()
{
motors.mode_selection(Mode::MIT_MODE, 10, 10, 10); // Params: Mode::operation mode, feedback cycle 1, 2, 3
Sleep(10); // Windows: Sleep(ms) Linux: sleep(s)
motors.write_mit(cur, vel, pos, kp, kd); // Params: vector of target current, vector of target velocity, vector of target position,
// vector of kp, vector of kd
}
固定速度(电流的大小应视情况而定)
目标位置和KP为0,其他不为0
#include <multi_motor_control.h>
vector<int16_t>cur = { 300, 300 };
vector<int16_t>vel = { 500, 500 };
vector<int32_t>pos = { 0, 0 };
vector<float>kp = { 0.0, 0.0 };
vector<float>kd = { 10.0, 10.0 };
vector<uint8_t>ids = { 0x01, 0x17 };
string port_name = "COM27"; // Windows: "COM*" Linux: "/dev/ttyUSB*"
MultiMotorControl motors(port_name, 921600, ids); // Params: serial port path (string), baud rate (uint32_t), actuator ids (0x05060100, 0x05061700)
int main()
{
motors.mode_selection(Mode::MIT_MODE, 10, 10, 10); // Params: Mode::operation mode, feedback cycle 1, 2, 3
Sleep(10); // Windows: Sleep(ms) Linux: sleep(s)
motors.write_mit(cur, vel, pos, kp, kd); // Params: vector of target current, vector of target velocity, vector of target position,
// vector of kp, vector of kd
}
点到点(电流的大小应视情况而定)
目标速度为0,其他不为0
#include <multi_motor_control.h>
vector<int16_t>cur = { 300, 300 };
vector<int16_t>vel = { 0, 0 };
vector<int32_t>pos = { 65535, 65535 };
vector<float>kp = { 13.1, 13.1 };
vector<float>kd = { 10.0, 10.0 };
vector<uint8_t>ids = { 0x01, 0x17 };
string port_name = "COM27"; // Windows: "COM*" Linux: "/dev/ttyUSB*"
MultiMotorControl motors(port_name, 921600, ids); // Params: serial port path (string), baud rate (uint32_t), actuator ids (0x05060100, 0x05061700)
int main()
{
motors.mode_selection(Mode::MIT_MODE, 10, 10, 10); // Params: Mode::operation mode, feedback cycle 1, 2, 3
Sleep(10); // Windows: Sleep(ms) Linux: sleep(s)
motors.write_mit(cur, vel, pos, kp, kd); // Params: vector of target current, vector of target velocity, vector of target position,
// vector of kp, vector of kd
}
Python
mode_selection(MotorControl object, MIT_MODE, xx, xx, xx)
write_mit(MotorControl object, [Target current], [Target velocity], [Target position], [KP], [KD])
demo: Point to Point
目标速度为0,其他不为0
from motor_control import *
id = [0x01, 0x17] # list of int
cur = [300, 300] # list of int
vel = [0, 0] # list of int
pos = [65535, 65535] # list of int
kp = [13.1, 13.1] # list of float
kd = [10.0, 10.0] # list of float
port_name = "COM27" # Windows: "COM*" Linux: "/dev/ttyUSB*"
motor = MotorControl(port_name, id, 921600) # Params: serial port (str), list of actuator ID (0x05060100, 0x05061700)
mode_selection(motor, MIT_MODE, 10, 10, 10) # Params: MotorControl object, operation mode, feedback cycle 1, 2, 3
time.sleep(0.01)
write_mit(motor, cur, vel, pos, kp, kd) # Params: MotorControl object, list of target current, list of target velocity, list of target position, list of KP, list of KD
Fixed speed (the current should be determined according to the situation)
The target position and KP are 0, and the others are not 0
from motor_control import *
id = [0x01, 0x17] # list of int
cur = [300, 300] # list of int
vel = [500, 500] # list of int
pos = [0, 0] # list of int
kp = [0.0, 0.0] # list of float
kd = [10.0, 10.0] # list of float
port_name = "COM27" # Windows: "COM*" Linux: "/dev/ttyUSB*"
motor = MotorControl(port_name, id, 921600) # Params: serial port (str), list of actuator ID (0x05060100, 0x05061700)
mode_selection(motor, MIT_MODE, 10, 10, 10) # Params: MotorControl object, operation mode, feedback cycle 1, 2, 3
time.sleep(0.01)
write_mit(motor, cur, vel, pos, kp, kd) # Params: MotorControl object, list of target current, list of target velocity, list of target position, list of KP, list of KD
Fixed torque (the current should be determined according to the situation)
The target current is not 0, and the others are 0
from motor_control import *
id = [0x01, 0x17] # list of int
cur = [500, 500] # list of int
vel = [0, 0] # list of int
pos = [0, 0] # list of int
kp = [0.0, 0.0] # list of float
kd = [0.0, 0.0] # list of float
port_name = "COM27" # Windows: "COM*" Linux: "/dev/ttyUSB*"
motor = MotorControl(port_name, id, 921600) # Params: serial port (str), list of actuator ID (0x05060100, 0x05061700)
mode_selection(motor, MIT_MODE, 10, 10, 10) # Params: MotorControl object, operation mode, feedback cycle 1, 2, 3
time.sleep(0.01)
write_mit(motor, cur, vel, pos, kp, kd) # Params: MotorControl object, list of target current, list of target velocity, list of target position, list of KP, list of KD